When to Text Again After the First Date
If you're but getting started with programming dates and times in Swift 5, you lot probably looked at Apple'south documentation and got dislocated. Let me reassure you that it isn't your fault. For starters, Apple'south documentation hasn't been very good lately, and I'm not the but developer who's noticed this refuse in quality.
At that place'southward as well the fact that working with dates and times in Swift 5 seems unnecessarily complicated. If you lot've come up to Swift from other programming languages, such as JavaScript, which uses a single object type calledAppointment
, the idea of having this set of classes just to handle dates and times looks similar overkill:
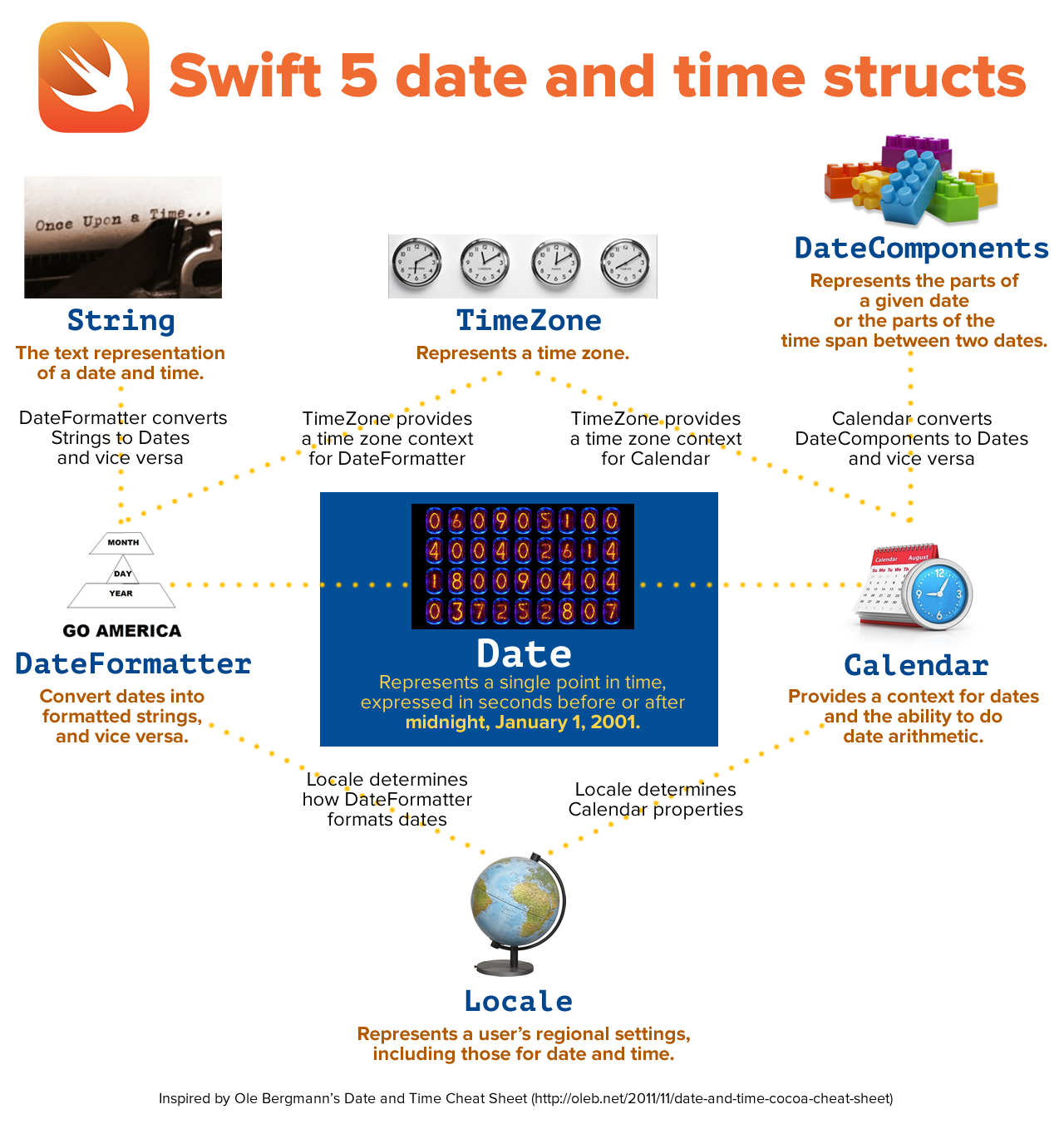
I promise you that there's a method to this madness. The set up of structs for working with dates and times in Swift 5 make for a super-flexible system that volition let you keep shop, calculate, and display dates and times no thing what time zone, calendar system, or language you lot apply.
For example, with Swift, I tin can easily schedule an engagement for the tertiary Wednesday of July at 3:30 p.m. Pacific and then display that date as information technology would announced in the Coptic Calendar system in Melbourne, Australia. If you had to do that in JavaScript, the but easy answer is "get away" (there are, of course, some less polite variations of that answer).
This series,Dates and times in Swift v, will aid you lot make sense of the set of classes that Swift provides for dealing with timekeeping and time calculations. It volition exercise so with a lot of examples and experimentation. I strongly recommend that you burn upwardly Xcode, open a Swift playground, and follow forth.
In this article, I'll show you the following:
- The
Date
struct, which stores date and times in Swift v. - The
Agenda
struct, which represents one of 16 different calendar systems, and provides a meaningful context forAppointment
due south. - The
DateComponents
struct, which a collection of engagement and time components, such as years, months, days, hours, minutes, and and so on. - How to create a
Date
object representing the current engagement and time. - How to create a
Date
object representing a given date and fourth dimension. - How to create a
Date
object based on criteria such as "the offset Fri of June 2020." - How to extract the parts of a date, such as the year, month, twenty-four hour period, time, and then on from a
Date
object.
The Date struct
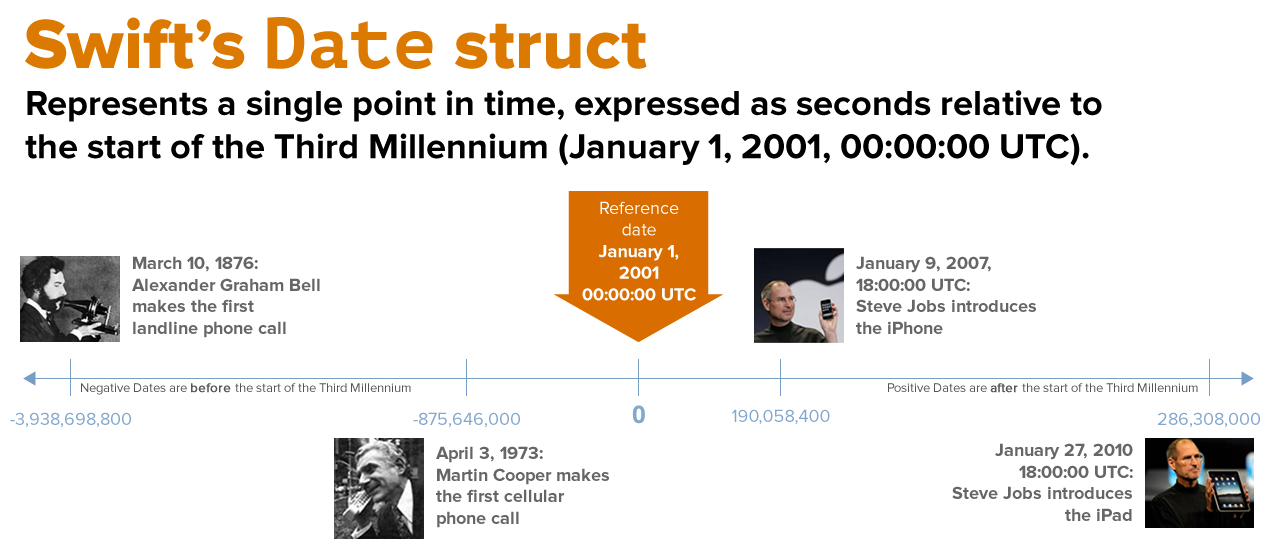
The Date
struct is used to correspond dates and times in Swift 5, and it'south designed to do and so in the about flexible way possible: equally a number of seconds relative to the showtime of the Third Millennium, January 1, 2001, 00:00:00 UTC.
This arroyo allows Date
to be independent of whatever time zone or any calendar system; y'all store it equally an corporeality of time before or after the start of the Third Millennium and use other objects (which you'll run across soon) to convert into the appropriate calendar, time zone, and format.
To create a Appointment
object containing the current engagement and fourth dimension, simply create an example of Appointment
using the default, no-argument initializer.
Open a playground in Xcode and add the following:
// To create a Date representing the electric current date and time, // simply initialize a new Date. let now = Date() // Contains the date and time that this object // was instantiated.
To come across the value stored inside the newly created Date
object, use Engagement
'south timeIntervalSinceReferenceDate
belongings, which contains the number of seconds since the reference date.
Add together the following to the playground, then run it:
// How many seconds accept passed betwixt the Date we just created // and the reference appointment of Jan 1, 2001, 00:00:00 UTC? print("It'due south been \(now.timeIntervalSinceReferenceDate) seconds since the start of the 3rd Millennium.")
When I ran that line of code (just before nine:00 a.thou. EDT on Tuesday, May 26, 2020), I got this output: Information technology's been 612190714.691352 seconds since the first of the Third Millennium.
To see the Date
's value in a more meaningful form, employ Engagement
's description
belongings to come across it in "YYYY-MM-DD HH:MM:SS +HHMM" format, or the clarification(with:)
method with a Locale
instance to display its value using a specified locale.
Add together the following to the playground, then run it:
// Use Date's description belongings for debugging impress(now.description) // YYYY-MM-DD HH:MM:SS +HHMM print(at present.description(with: Locale(identifier: "en-U.s.a."))) // US English print(now.description(with: Locale(identifier: "en-GB"))) // UK English* impress(now.description(with: Locale(identifier: "es"))) // Spanish impress(at present.clarification(with: Locale(identifier: "zh-Hans"))) // Simplified Chinese // * Yes, I know that "gb" ways "Swell United kingdom" and that information technology'southward not the same matter // every bit the United kingdom of great britain and northern ireland. I didn't come up up with these locale names!
To create a Date
object containing a date and time that isn't the current date and fourth dimension, and without other helper objects, you have to apply i of the following initializers:
Date initializer | What it does |
Date(timeIntervalSinceNow:) | Creates a Parameters:
|
Date(timeIntervalSinceReferenceDate:) | Creates a Parameters:
|
Engagement(timeIntervalSince1970:) | Creates a Parameters:
|
Date(timeInterval:since:) | Creates a Parameters:
|
Hither are some examples showing these initializers in action — add the following to the playground, then run it:
// Date's other initializers expect arguments of type TimeInterval, // which is a typealias for Double. // To create a Date a specified number of seconds before or after // the current date and fourth dimension, utilise the "timeIntervalSinceNow" initializer. let fiveMinutesAgo = Date(timeIntervalSinceNow: -five * threescore) let fiveMinutesFromNow = Date(timeIntervalSinceNow: v * threescore) // Martin Cooper made the outset cellular telephone telephone call on Apr iii, 1973. // We don't know the exact time the call was made, merely information technology happened // sometime during concern hours in New York City, in the U.Southward. Eastern // Fourth dimension Zone (UTC-5). // // Let's suppose that he made the call at 12:00 p.m.. That would hateful that // he fabricated the call 875,602,800 seconds Earlier the reference date and time. let firstCellularCallDate = Date(timeIntervalSinceReferenceDate: -875_602_800) // The "Stevenote" where the iPhone was introduced started on January 9, 2007, // 10 a.k. Pacific time (UTC-7), 190,058,400 seconds AFTER the reference date and time. let iPhoneStevenoteDate = Date(timeIntervalSinceReferenceDate: 190_058_400) // Unix time (a.chiliad.a. POSIX time or Epoch Fourth dimension) is the way time is represented // by Unix, Unix-like, and other operating systems. It defines time as a // number of seconds later on the Unix Epoch, January 1, 1970, 00:00:00 UTC. // To create a Appointment relative to the Unix Epoch, utilize the // "timeIntervalSince1970" initializer. let oneYear = TimeInterval(sixty * sixty * 24 * 365) let newYears1971 = Date(timeIntervalSince1970: oneYear) let newYears1969 = Engagement(timeIntervalSince1970: -oneYear) // To create a Date relative to another Date, use the // "timeInterval:Since:" initializer. // // The "Stevenote" where the iPad was introduced started on January 27, 2010, // x a.yard. Pacific time (UTC-vii), 96,249,600 seconds afterward the start of the // iPhone Stevenote three years earlier. let secondsBetweeniPhoneAndiPadStevenote = TimeInterval(96_249_600) permit iPadStevenoteDate = Date(timeInterval: secondsBetweeniPhoneAndiPadStevenote, since: iPhoneStevenoteDate)
Of form, nosotros don't think of dates and times in terms of seconds relative to the start of the 3rd Millennium, or the start of the Unix Epoch, or any other arbitrary date and time. That's why dates and times in Swift 5 brand utilize of a couple of other structs to help the states make sense of Engagement
s:Calendar
andDateComponents
. Calendar
southward requite dates context, and DateComponents
allow united states of america gather dates or break dates apart.
The Calendar struct
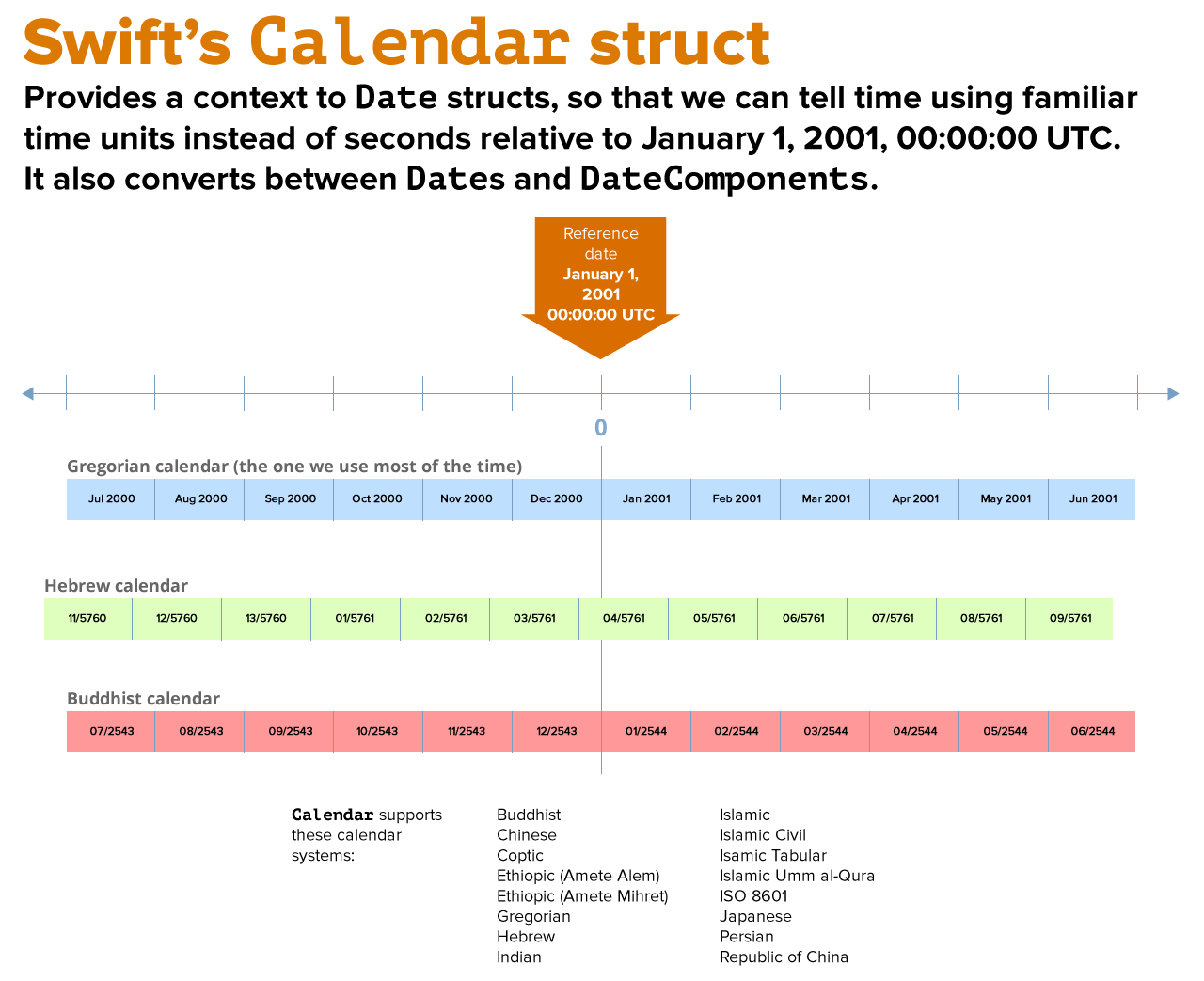
Call back of theCalendar
struct every bit a manner to viewEngagement
s in a way that makes more sense to u.s.: non as a number of seconds before or subsequently January 1, 2001 00:00:00 UTC, but in terms of a date in a calendar organization.
TheCalendar
struct supports 16 dissimilar agenda systems, including the Gregorian calendar (a.k.a. the Western or Christian agenda), which is likely the i y'all utilize the most.
The DateComponents struct
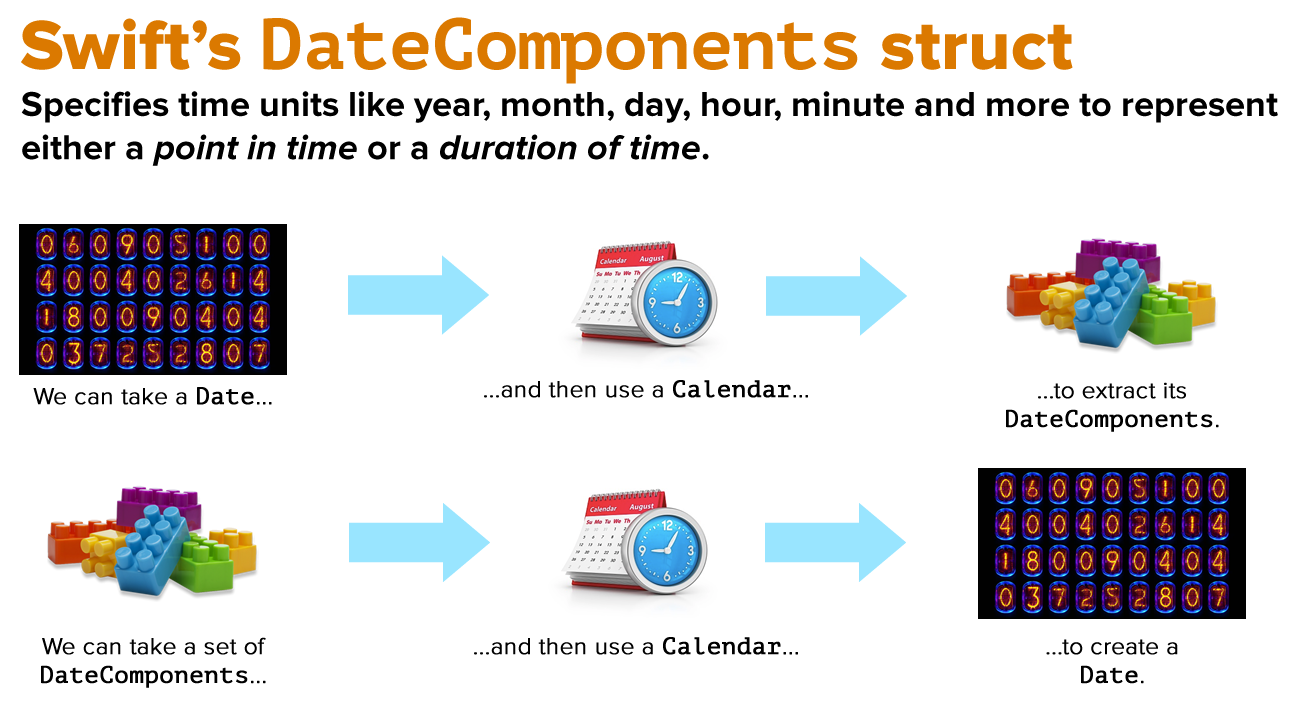
The DateComponents
struct allows united states to assemble a point in time or a length of time out of components such as year, month, mean solar day, hr, infinitesimal, and more. We'll use DateComponents
to construct a Date
object using a twelvemonth, month, day and time, and also deconstruct a Date
object into a year, calendar month, day and time.
Consider Swift's reference appointment: January i, 2001 at 00:00:00 UTC. Here are what its components are in various agenda systems:
Calendar | Engagement components for January 1, 2001 00:00:00 UTC |
Gregorian |
|
Hebrew |
|
Buddhist |
|
Let'south employ Agenda
and DateComponents
to get in easier to create Dates
.
Creating known Date
s with Calendar
andDateComponents
Let's create a Date
based on the first moment in phone history: March 10, 1876, when Alexander Graham Bell fabricated the get-go telephone telephone call. I don't know what time he made the call on that mean solar day, then for this instance, I'm going to presume that it happened at 1:00 p.m..
Add the post-obit to the playground, then run it:
// 1 // To translate between Dates and DateComponents, you demand a Calendar. // The user's Calendar is probably the one you'll use nearly ofttimes, // as information technology incorporates the user'southward locale and time zone settings. let userCalendar = Calendar.electric current // March 10, 1876: The day Alexander Graham Bell // made the first telephone call // --------------------------------------------- // 2 // DateComponents' full initializer method is very thorough, merely very long. let firstLandLineCallDateComponents = DateComponents( agenda: nil, timeZone: TimeZone(secondsFromGMT: -18000), era: nil, year: 1876, calendar month: 3, day: 10, hour: xiii, minute: 00, second: 00, nanosecond: cypher, weekday: nix, weekdayOrdinal: nil, quarter: nil, weekOfMonth: cipher, weekOfYear: aught, yearForWeekOfYear: nil) // 3 allow firstLandLineCallDate = userCalendar.appointment(from: firstLandLineCallDateComponents)! // 4 impress("The start land line phone call happened on \(firstLandLineCallDate.clarification(with: Locale(identifier: "en-United states of america"))).") impress("That's \(firstLandLineCallDate.timeIntervalSinceReferenceDate) seconds since the start of the Third Millennium.")
In the lawmaking, nosotros:
- Become the user'southward current
Calendar
. - Create a
DateComponents
struct,firstLandLineCallDateComponents
, providing values for the timeZone: ,year:
,month:
, andday:
,hour:
,minute:
, and2d:
parameters, andnil
for all the others. Bell fabricated the phone call in his laboratory in Boston, whose time zone is UTC-v. Nosotros construct the fourth dimension zone using theTimeZone(secondsFromGMT:)
constructor, which specifies how many seconds the given time zone is ahead or backside UTC (GMT — Greenwich Elevation Time — is the onetime name for UTC). - Use the user's
Agenda
to createfirstLandLineCallDate
usingfirstLandLineCallDateComponents
. - Print the date of the call using the American English format besides as in terms of seconds since the start of the Third Millennium. Since the call happened over a century before the Third Millennium, this number is negative.
On my calculator, the output is:
The start land line phone phone call happened on Friday, March x, 1876 at 12:03:58 PM GMT-04:56:02.
That's -3938655600.0 seconds since the start of the Third Millennium.
Let'southward create another engagement: December 9, 1968 at three:45 p.m. Pacific, when Douglas Englebart changes the globe of computing with his demonstration of the GUI, mouse, chording keyboard, hypertext links, and collaborative document editing — a session that would come up to exist called "The Mother of All Demos."
Add the following to the playground, then run it:
// December 9, 1968, 3:45 p.m. Pacific Time: // Douglas Englebart gives "The Female parent of All Demos" // ------------------------------------------------- // This fourth dimension, we'll use only the DateComponents initializer parameters nosotros need. // 1 allow motherOfAllDemosDateComponents = DateComponents( timeZone: TimeZone(identifier: "America/Los_Angeles"), year: 1968, month: 12, day: 9, hour: 15, minute: 45 ) // ii let motherOfAllDemosDate = userCalendar.engagement(from: motherOfAllDemosDateComponents)! // 3 impress("The Mother of All Demos happened on \(motherOfAllDemosDate.clarification(with: Locale(identifier: "en-US"))).") impress("That's \(motherOfAllDemosDate.timeIntervalSince1970) seconds since the commencement of the Unix Epoch.")
In the code, we:
- Create a
DateComponents
struct,motherOfAllDemosDateComponents
, providing values for merely the parameters that matter t u.s.a.:timeZone:
,year:
,month:
, andtwenty-four hour period:
,hour:
, andminute:
. This fourth dimension, nosotros construct the time zone using theTimeZone(identifier:)
constructor, which specifies the time zone by the advisable TZ database name. - Use the user's
Calendar
(userCalendar
, which we've already alleged) to createmotherOfAllDemosDate
usingmotherOfAllDemosDateComponents
. - Print the engagement of the call using the American English format as well as in terms of seconds since the get-go of the Unix Epoch. Since the call happened a niggling over a year before the Unix Epoch, this number is negative.
On my estimator, the output is:
The Mother of All Demos happened on Monday, December 9, 1968 at half dozen:45:00 PM GMT-05:00.
That'south -33437700.0 seconds since the start of the Unix Epoch.
Permit's endeavour creating another momentous date in phone history:the twenty-four hours when Martin Cooper made the start cellular phone phone call, April 3, 1973.
Add the post-obit to the playground, then run it:
// April 3, 1973: Martin Cooper makes the start // cellular telephone telephone call // -------------------------------------------- // ane var firstCellCallDateComponents = DateComponents() // two firstCellCallDateComponents.year = 1973 firstCellCallDateComponents.month = four firstCellCallDateComponents.twenty-four hour period = iii firstCellCallDateComponents.timeZone = TimeZone(abbreviation: "EST") // three permit firstCellCallDate = userCalendar.date(from: firstCellCallDateComponents)! print("Martin Cooper made the commencement cellular call on \(firstCellCallDate.description(with: Locale(identifier: "en-US"))).") print("That's \(firstCellCallDate.timeIntervalSince(motherOfAllDemosDate)) seconds since the Mother of All Demos.")
In the code, we:
- Create an empty
DateComponents
struct,firstCellCallDateComponents
. Note that we're usingvar
instead ofpermit
to declare information technology; that'southward considering we're going to set its backdrop after it'south been declared, and y'all can't exercise that to a struct alleged withlet
. - Set the year, month, and day properties of
firstCellCallDateComponents
to correspond to the engagementApril 3, 1973 in Eastern Standard Time. This time, we construct the time zone using theTimeZone(abbreviation:)
constructor, which specifies the time zone by the appropriate fourth dimension zone abbreviation. - Use the user'southward
Calendar
to createfirstCellCallDate
usingfirstCellCallDateComponents
.
On my computer, the output is:
Martin Cooper made the first cellular telephone call on Tuesday, Apr three, 1973 at 12:00:00 AM Eastern Standard Time.
That's 136098900.0 seconds since the Female parent of All Demos.
Note that in the absenteeism of a specified time, the assumed time is 00:00:00.
Discovering Date
s with Calendar
andDateComponents
So far, we've created Date
s based on known dates and times — March ten, 1876, Dec nine, 1968, and April 3, 1973. How near Date
s where we don't have a specific date, merely have enough criteria to specify a appointment? The keen thing most working with dates and times in Swift 5 is that the Calendar
class is that it does its best to work with theDateComponents
that yous give information technology, andDateComponents
gives you all sorts of means to specify a date.
Having come from Canada, the country with the world'due south highest per capita donut shop concentration and the people who consume the most donuts per capita,I can assure y'all that National Donut Solar day has been a real thing since 1938, and it takes place on the first Friday in June. We tin can find out what date it falls on in 2022 — or any other year — through the judicious use of DateComponents
properties.
Add the post-obit to the playground, and so run it:
// The first Friday in June, 2020: // National Donut Mean solar day // ------------------------------- permit donutDay2020Components = DateComponents( year: 2020, // Nosotros want a date in 2020, month: half-dozen, // in June. weekday: vi, // We want a Fri; weekdayOrdinal: ane // the beginning one. ) let donutDay2020 = userCalendar.date(from: donutDay2020Components)! print("Donut Solar day 2022 happens on \(donutDay2020Date.description(with: Locale(identifier: "en-United states of america"))).")
Y'all should be familiar with theyear
andmonth
DayComponents
properties by now, and we're using a couple that may be new to yous:
-
weekday
: Specifies a day of the week. With the Gregorian agenda, valid values are 1 through 7, where 1 is Lord's day, 2 is Monday, 3 is Tuesday, and and then on. Since nosotros're looking for a Friday, nosotros've set this value to half-dozen. -
weekdayOrdinal
: Specifies the guild of the given weekday in the adjacent larger specified agenda unit. Since nosotros fixweekday
to 6 and set this value to 1, and since the next largest specified agenda unit wasmonth
, we'll go the engagement of the first Friday of the calendar month.
On my computer, the output is:
Donut Day 2022 happens on Fri, June five, 2022 at 12:00:00 AM Eastern Daylight Fourth dimension.
Note that in the absence of a specified time zone, the assumed time zone is the organisation time zone, which in my case is Eastern Daylight Time (UTC-iv).
In the spirit of the fake book cover shown above, let's run into what happens when we use Donut Day 2020'southward components, except for the calendar month.
Add the following to the playground, then run it:
// Mystery Friday 2022 // ------------------------------- permit mysteryFridayDateComponents = DateComponents( year: 2020, // Nosotros want a date in 2020: weekday: vi, // A Friday; weekdayOrdinal: i // the beginning one. ) permit mysteryFridayDate = userCalendar.appointment(from: mysteryFridayDateComponents)! print("Mystery Friday happens on \(mysteryFridayDate.description(with: Locale(identifier: "en-US"))).")
On my estimator, the output is:
Mystery Fri happens on Friday, January iii, 2022 at 12:00:00 AM Eastern Standard Time.
That makes sense: By specifying year 2020, weekday 6 and weekday ordinal 1, we merely asked for the first Friday of the year.
Suppose you're meeting up with a friend in Tokyo some relaxing Suntory times at 9:00 p.k. on the Thursday of the 27th week of 2020. What is that date?
The reply comes from this lawmaking:
// 9:00 p.m. Tokyo fourth dimension on the Thursday of the 27th week of 2022 // ------------------------------------------------------------- var relaxingSuntoryTimesDateComponents = DateComponents( timeZone: TimeZone(identifier: "Asia/Tokyo")!, year: 2020, 60 minutes: 21, weekday: v, weekOfYear: 27 ) permit relaxingSuntoryTimesDate = userCalendar.appointment(from: relaxingSuntoryTimesDateComponents)! print("Th on the 27th week of 2022 at ix:00 p.one thousand. Tokyo time is \(relaxingSuntoryTimesDate.description(with: Locale(identifier: "en-US"))).")
On my computer, the output is:
Thursday on the 27th week of 2022 at 9:00 p.m. Tokyo time is Thursday, July 2, 2022 at 8:00:00 AM Eastern Daylight Fourth dimension.
Here'south a uncomplicated question: What's the 234th twenty-four hour period of 2020?
Add together the following to the playground, and then run information technology:
// 234th 24-hour interval of 2022 // ----------------- permit day234DateComponents = DateComponents( year: 2020, // We desire a date in 2020: day: 234 // the 234th day. ) permit day234Date = userCalendar.date(from: day234DateComponents)! print("The 234th day of 2022 is \(day234Date.clarification(with: Locale(identifier: "en-US"))).")
On my estimator, the output is:
The 234th day of 2022 is Friday, August 21, 2022 at 12:00:00 AM Eastern Daylight Time.
The "10 Thou 60 minutes Rule", which states that it takes 10,000 hours of directed practice to become an good at something, was popularized by Malcolm Gladwell in his novel Blink. At that place's been a lot of debate about the truth of the dominion, and we're going to side-step it to inquire a related question: If I set up out to get ten,000 hours of non-stop exercise starting on midnight of January 1, 2020, when would I exist done?
Add the following to the playground, then run it:
// 10000th hour of 2022 // ----------------- let hour10kDateComponents = DateComponents( yr: 2020, // We want a date in 2020: hour: 10000 // the 10000th hour. ) permit hour10kDate = userCalendar.date(from: hour10kDateComponents)! print("Your x,000 hours would consummate on \(hour10kDate.description(with: Locale(identifier: "en-The states"))).")
On my figurer, the output is:
Your x,000 hours would complete on Saturday, February 20, 2022 at four:00:00 PM Eastern Standard Fourth dimension.
In other words, 10,000 hours is longer than a year.
Let'due south expect at the case of overflow. What happens if you endeavor to create a Date
using components that would define the nonsense date September 50th, 2020?
Add together the post-obit to the playground, then run it:
// September 50, 2022 // ------------------ var sept50DateComponents = DateComponents( year: 2020, month: 9, twenty-four hours: fifty) allow sept50Date = userCalendar.date(from: sept50DateComponents)! print("September fifty, 2022 is actually \(sept50Date.clarification(with: Locale(identifier: "en-U.s.a."))).")
On my computer, the output is:
September 50, 2022 is really Tuesday, October xx, 2022 at 12:00:00 AM Eastern Daylight Time.
Swift treats these appointment components as "the first of September, plus 50 days" — September's 30 days, plus an boosted twenty days into Oct.
Let's extractDateComponents
from aDate
, part one
Now that we've created someAppointment
s usingDateComponents
, permit's practise the reverse and excerptDateComponents
from givenDate
south. We'll continue with our playground and use aDate
we've already created
Allow's extract the year, month, and day from firstLandPhoneCallDate
, which corresponds to the date of Alexander Graham Bell's historic phone phone call, March 10, 1876:
// We desire to extract the year, month, and day from firstLandLineCallDate let alexanderGrahamBellDateComponents = userCalendar.dateComponents([.year, .month, .solar day], from: firstLandLineCallDate) impress("The commencement land line telephone call happened \(-firstLandLineCallDate.timeIntervalSinceNow) seconds ago.") print("Year: \(alexanderGrahamBellDateComponents.twelvemonth!)") impress("Month: \(alexanderGrahamBellDateComponents.month!)") impress("Mean solar day: \(alexanderGrahamBellDateComponents.twenty-four hours!)")
When I ran it on my computer, the output was:
The start land line phone call happened 4550860374.044979 seconds ago.
Twelvemonth: 1876
Month: 3
Twenty-four hour period: x
Let's extractDateComponents
from aDate
, part ii
This time, allow's extract the DateComponents
from some other Date
we'd previously divers: The date and time of the "Stevenote" where the original iPhone was commencement announced:
Information technology happened 190,058,400 seconds later on the reference date. For most of united states, this is a meaningless figure, so we'll extract the following DateComponents
from thisEngagement
:
- Year
- Calendar month
- 24-hour interval
- Hr
- Minute
- What day of the week it fell on
- What week of the year it savage on
Here's the code:
// The original iPad Stevenote (x a.thousand. Pacific fourth dimension, // Jan 27, 2010) // ---------------------------------------------------- // We desire to extract ALL the DateComponents for this appointment // in local time for the issue (which took identify in San Francisco). let iPadStevenoteDateComponents = pacificCalendar.dateComponents([.calendar, .day, .era, .hour, .minute, .calendar month, .nanosecond, .quarter, .second, .timeZone, .weekday, .weekdayOrdinal, .weekOfMonth, .weekOfYear, .twelvemonth, .yearForWeekOfYear], from: iPadStevenoteDate) print("The original iPad Stevenote happened \(-iPadStevenoteDate.timeIntervalSinceNow) seconds ago.") print("Calendar: \(iPadStevenoteDateComponents.calendar!.identifier)") print("Day: \(iPadStevenoteDateComponents.solar day!)") print("Era: \(iPadStevenoteDateComponents.era!)") print("60 minutes: \(iPadStevenoteDateComponents.hour!)") print("Minute: \(iPadStevenoteDateComponents.minute!)") print("Month: \(iPadStevenoteDateComponents.month!)") print("Nanosecond: \(iPadStevenoteDateComponents.nanosecond!)") print("Quarter: \(iPadStevenoteDateComponents.quarter!)") print("2d: \(iPadStevenoteDateComponents.2nd!)") print("Fourth dimension zone: \(iPadStevenoteDateComponents.timeZone!)") impress("Weekday: \(iPadStevenoteDateComponents.weekday!)") impress("Weekday ordinal: \(iPadStevenoteDateComponents.weekdayOrdinal!)") impress("Calendar week of calendar month: \(iPadStevenoteDateComponents.weekOfMonth!)") print("Week of yr: \(iPadStevenoteDateComponents.weekOfYear!)") print("Year: \(iPadStevenoteDateComponents.year!)") print("Twelvemonth for week of year: \(iPadStevenoteDateComponents.yearForWeekOfYear!)")
Let's extractDateComponents
from aDate
, part iii
Permit'southward effort it once more with another key iOS date — the Stevenote where the original iPad was announced:
This time, if yous were to ask Swift when this Stevenote took place, information technology would reply "286,308,000 seconds after the reference engagement". Allow's getall theDateComponents
for this date:
// The original iPad Stevenote (x a.one thousand. Pacific time, // January 27, 2010) // ---------------------------------------------------- // We want to extract ALL the DateComponents for this engagement // in local time for the outcome (which took place in San Francisco). let iPadStevenoteDateComponents = pacificCalendar.dateComponents([.calendar, .day, .era, .hour, .minute, .month, .nanosecond, .quarter, .2d, .timeZone, .weekday, .weekdayOrdinal, .weekOfMonth, .weekOfYear, .yr, .yearForWeekOfYear], from: iPadStevenoteDate) print("The original iPad Stevenote happened \(-iPadStevenoteDate.timeIntervalSinceNow) seconds ago.") print("Agenda: \(iPadStevenoteDateComponents.agenda!.identifier)") print("Day: \(iPadStevenoteDateComponents.24-hour interval!)") print("Era: \(iPadStevenoteDateComponents.era!)") print("Hour: \(iPadStevenoteDateComponents.hour!)") print("Infinitesimal: \(iPadStevenoteDateComponents.infinitesimal!)") print("Calendar month: \(iPadStevenoteDateComponents.month!)") print("Nanosecond: \(iPadStevenoteDateComponents.nanosecond!)") print("Quarter: \(iPadStevenoteDateComponents.quarter!)") print("Second: \(iPadStevenoteDateComponents.second!)") print("Time zone: \(iPadStevenoteDateComponents.timeZone!)") print("Weekday: \(iPadStevenoteDateComponents.weekday!)") print("Weekday ordinal: \(iPadStevenoteDateComponents.weekdayOrdinal!)") print("Week of month: \(iPadStevenoteDateComponents.weekOfMonth!)") impress("Week of twelvemonth: \(iPadStevenoteDateComponents.weekOfYear!)") print("Year: \(iPadStevenoteDateComponents.year!)") print("Year for week of year: \(iPadStevenoteDateComponents.yearForWeekOfYear!)")
When I ran it on my computer, the output was:
The original iPad Stevenote happened 325902655.723246 seconds ago.
Calendar: gregorian
Day: 27
Era: 1
Hour: ten
Minute: 0
Calendar month: 1
Nanosecond: 0
Quarter: 0
Second: 0
Time zone: America/Los_Angeles (fixed)
Weekday: 4
Weekday ordinal: 4
Week of month: 5
Week of twelvemonth: v
Year: 2010
Yr for week of twelvemonth: 2010
Let's take a await at eachDateComponents
belongings and what it represents:
Property | Description |
---|---|
calendar | The agenda system for the date represented by this set ofDateComponents . We got theseDateComponents by converting aAppointment using a Gregorian Calendar, so in this case, this value isgregorian. |
day | The day number of this particular date and time. For January 27, 2010, 18:00:00 UTC, this value is27. |
era | The era for this particular appointment, which depends on the date'due south agenda organisation. In this case, we're using the Gregorian calendar, which has 2 eras:
|
hr | The hour number of this detail engagement and time. For January 27, 2010, xviii:00:00 UTC, this value is13, because in my time zone, 18:00:00 UTC is xiii:00:00. |
infinitesimal | The infinitesimal number of this particular date and time. For January 27, 2010, 18:00:00 UTC, this value is0. |
month | The month number of this particular date and time. For January 27, 2010, 18:00:00 UTC, this value isi. |
nanosecond | The nanosecond number of this particular engagement and fourth dimension. For January 27, 2010, xviii:00:00 UTC, this value is0. |
quarter | The quarter number of this particular date and time. January 27, 2010, 18:00:00 UTC, is in the outset quarter of the year, and so this value is0. |
2d | The second number of this particular date and time. For January 27, 2010, xviii:00:00 UTC, this value is0. |
timeZone | The fourth dimension zone of this item appointment and time. I'grand in the UTC-five time zone (US Eastern), and then this value is set up to that time zone. |
weekday | The day of the week of this item date and time. In the Gregorian calendar, Sunday is 1, Mon is 2, Tuesday is iii, and and so on. Jan 27, 2010, was a Midweek, then this value is4. |
weekdayOrdinal | The position of the weekday within the adjacent larger specified calendar unit, which in this case is a month. So this specifiesnthursday weekday of the given month. Jauary 27, 2010 was on the quaternary Wednesday of the calendar month, so this value is4. |
weekOfMonth | The week of the month of this particular date and time. January 27, 2010 fell on the fifth week of Jan 2010, so this value is5. |
weekOfYear | The calendar week of the year of this particular date and time. January 27, 2010 vicious on the 5th week of 2010, so this value is5. |
twelvemonth | The year number of this particular appointment and time. For Jan 27, 2010, eighteen:00:00 UTC, this value is2010. |
yearForWeekOfYear | Oh wow, this is so hard to explain that I'll leave it to Apple's docs. |
Wrapping it all upward
Here'southward the complete code for the playground containing all the code we only worked with while learning about dates and times in Swift 5:
import UIKit // To create a Date representing the current date and time, // merely initialize a new Appointment. let now = Date() // Contains the date and fourth dimension that this object // was instantiated. // How many seconds have passed between the Date nosotros just created // and the reference engagement of January ane, 2001, 00:00:00 UTC? impress("It'southward been \(now.timeIntervalSinceReferenceDate) seconds since the start of the Third Millennium.") // Use Date's description holding for debugging print(now.clarification) // YYYY-MM-DD HH:MM:SS +HHMM impress(now.clarification(with: Locale(identifier: "en-U.s."))) // U.s.a. English print(now.description(with: Locale(identifier: "en-GB"))) // UK English* print(at present.clarification(with: Locale(identifier: "es"))) // Castilian print(now.description(with: Locale(identifier: "zh-Hans"))) // Simplified Chinese // * Yes, I know that "gb" means "Cracking U.k." and that it's non the same thing // as the Great britain. I didn't come upwardly with these locale names! // Date's other initializers expect arguments of type TimeInterval, // which is a typealias for Double. // To create a Engagement a specified number of seconds earlier or later // the current appointment and time, employ the "timeIntervalSinceNow" initializer. let fiveMinutesAgo = Appointment(timeIntervalSinceNow: -5 * sixty) let fiveMinutesFromNow = Date(timeIntervalSinceNow: v * 60) // Martin Cooper made the first cellular telephone call on April 3, 1973. // We don't know the verbal fourth dimension the call was made, merely information technology happened // one-time during business hours in New York City, in the U.Southward. Eastern // Time Zone (UTC-5). // // Permit'due south suppose that he made the phone call at 12:00 p.yard.. That would mean that // he made the phone call 875,602,800 seconds Earlier the reference date and time. permit firstCellularCallDate = Appointment(timeIntervalSinceReferenceDate: -875_602_800) // The "Stevenote" where the iPhone was introduced started on January 9, 2007, // 10 a.m. Pacific time (UTC-seven), 190,058,400 seconds AFTER the reference date and time. let iPhoneStevenoteDate = Appointment(timeIntervalSinceReferenceDate: 190_058_400) // Unix time (a.chiliad.a. POSIX fourth dimension or Epoch Time) is the fashion time is represented // by Unix, Unix-like, and other operating systems. It defines time as a // number of seconds after the Unix Epoch, January 1, 1970, 00:00:00 UTC. // To create a Date relative to the Unix Epoch, apply the // "timeIntervalSince1970" initializer. let oneYear = TimeInterval(60 * 60 * 24 * 365) let newYears1971 = Date(timeIntervalSince1970: oneYear) let newYears1969 = Engagement(timeIntervalSince1970: -oneYear) // To create a Date relative to another Date, use the // "timeInterval:Since:" initializer. // // The "Stevenote" where the iPad was introduced started on January 27, 2010, // ten a.m. Pacific time (UTC-7), 96,249,600 seconds later on the get-go of the // iPhone Stevenote three years before. let secondsBetweeniPhoneAndiPadStevenote = TimeInterval(96_249_600) let iPadStevenoteDate = Date(timeInterval: secondsBetweeniPhoneAndiPadStevenote, since: iPhoneStevenoteDate) // To translate between Dates and DateComponents, you lot need a Calendar. // The user's Calendar is probably the one y'all'll use most often, // as it incorporates the user's locale and time zone settings. let userCalendar = Calendar.current // March ten, 1876: The mean solar day Alexander Graham Bell // made the starting time phone phone call in Boston (UTC-5) // --------------------------------------------- // DateComponents' full initializer method is very thorough, simply very long. let firstLandLineCallDateComponents = DateComponents( agenda: nil, timeZone: TimeZone(secondsFromGMT: -18000), era: zero, year: 1876, month: iii, day: x, hr: 12, minute: 00, second: 00, nanosecond: nil, weekday: nix, weekdayOrdinal: nil, quarter: nil, weekOfMonth: naught, weekOfYear: nil, yearForWeekOfYear: zero) allow firstLandLineCallDate = userCalendar.date(from: firstLandLineCallDateComponents)! print("The first state line phone telephone call happened on \(firstLandLineCallDate.description(with: Locale(identifier: "en-US"))).") print("That's \(firstLandLineCallDate.timeIntervalSinceReferenceDate) seconds since the start of the Third Millennium.") // December nine, 1968, iii:45 p.m. Pacific Time: // Douglas Englebart gives "The Mother of All Demos" // ------------------------------------------------- // This fourth dimension, we'll use but the DateComponents initializer parameters we demand. allow motherOfAllDemosDateComponents = DateComponents( timeZone: TimeZone(identifier: "America/Los_Angeles"), yr: 1968, month: 12, mean solar day: ix, 60 minutes: 15, minute: 45 ) let motherOfAllDemosDate = userCalendar.date(from: motherOfAllDemosDateComponents)! print("The Female parent of All Demos happened on \(motherOfAllDemosDate.description(with: Locale(identifier: "en-US"))).") print("That'south \(motherOfAllDemosDate.timeIntervalSince1970) seconds since the showtime of the Unix Epoch.") // April three, 1973: Martin Cooper makes the first // cellular phone call // -------------------------------------------- var firstCellCallDateComponents = DateComponents() firstCellCallDateComponents.year = 1973 firstCellCallDateComponents.month = four firstCellCallDateComponents.mean solar day = three firstCellCallDateComponents.timeZone = TimeZone(abbreviation: "EST") let firstCellCallDate = userCalendar.appointment(from: firstCellCallDateComponents)! impress("Martin Cooper made the beginning cellular call on \(firstCellCallDate.description(with: Locale(identifier: "en-U.s."))).") print("That'due south \(firstCellCallDate.timeIntervalSince(motherOfAllDemosDate)) seconds since the Female parent of All Demos.") // The showtime Friday in June, 2020: // National Donut Day // ------------------------------- let donutDay2020DateComponents = DateComponents( year: 2020, // We want a date in 2020, month: 6, // in June. weekday: 6, // We want a Fri; weekdayOrdinal: 1 // the first one. ) permit donutDay2020Date = userCalendar.engagement(from: donutDay2020DateComponents)! print("Donut Day 2022 happens on \(donutDay2020Date.clarification(with: Locale(identifier: "en-US"))).") // Mystery Fri 2022 // ------------------------------- permit mysteryFridayDateComponents = DateComponents( year: 2020, // We want a engagement in 2020: weekday: 6, // A Friday; weekdayOrdinal: 1 // the first one. ) let mysteryFridayDate = userCalendar.engagement(from: mysteryFridayDateComponents)! print("Mystery Friday happens on \(mysteryFridayDate.description(with: Locale(identifier: "en-Us"))).") // 9:00 p.thou. Tokyo fourth dimension on the Thursday of the 27th week of 2022 // ------------------------------------------------------------- var relaxingSuntoryTimesDateComponents = DateComponents( timeZone: TimeZone(identifier: "Asia/Tokyo")!, twelvemonth: 2020, 60 minutes: 21, weekday: 5, weekOfYear: 27 ) let relaxingSuntoryTimesDate = userCalendar.date(from: relaxingSuntoryTimesDateComponents)! impress("Thursday on the 27th week of 2022 at nine:00 p.k. Tokyo time is \(relaxingSuntoryTimesDate.description(with: Locale(identifier: "en-US"))).") // 234th twenty-four hour period of 2022 // ----------------- let day234DateComponents = DateComponents( year: 2020, // Nosotros want a date in 2020: 24-hour interval: 234 // the 234th day. ) allow day234Date = userCalendar.appointment(from: day234DateComponents)! impress("The 234th day of 2022 is \(day234Date.description(with: Locale(identifier: "en-US"))).") // 10000th hr of 2022 // ----------------- allow hour10kDateComponents = DateComponents( year: 2020, // We want a date in 2020: hr: 10000 // the 10000th 60 minutes. ) let hour10kDate = userCalendar.engagement(from: hour10kDateComponents)! impress("Your 10,000 hours would consummate on \(hour10kDate.description(with: Locale(identifier: "en-United states of america"))).") // September 50, 2022 // ------------------ var sept50DateComponents = DateComponents( year: 2020, month: 9, mean solar day: 50) permit sept50Date = userCalendar.date(from: sept50DateComponents)! print("September 50, 2022 is actually \(sept50Date.description(with: Locale(identifier: "en-Usa"))).") // Date of the commencement land line phone telephone call (March 10, 1876) // ------------------------------------------------------- // We want to extract the year, month, and day permit alexanderGrahamBellDateComponents = userCalendar.dateComponents([.year, .calendar month, .day], from: firstLandLineCallDate) impress("The kickoff land line phone phone call happened \(-firstLandLineCallDate.timeIntervalSinceNow) seconds ago.") print("Year: \(alexanderGrahamBellDateComponents.year!)") impress("Month: \(alexanderGrahamBellDateComponents.month!)") impress("Day: \(alexanderGrahamBellDateComponents.twenty-four hour period!)") // The original iPhone Stevenote (ten a.m. Pacific fourth dimension, // January ix, 2007) // ---------------------------------------------------- // We want to extract the year, month, 24-hour interval, hr, and minute from this date, // in local time for the effect (which took place in San Francisco). // Nosotros too want to know what day of the week and calendar week of the twelvemonth // this date fell on. // Create a calendar ready to Pacific standard time (UTC-8) var pacificCalendar = Calendar(identifier: .gregorian) pacificCalendar.timeZone = TimeZone(abbreviation: "PST")! allow iPhoneStevenoteDateComponents = pacificCalendar.dateComponents([.yr, .month, .day, .hour, .minute, .weekday, .weekOfYear], from: iPhoneStevenoteDate) impress("The original iPhone Stevenote happened \(-iPhoneStevenoteDate.timeIntervalSinceNow) seconds ago.") print("Year: \(iPhoneStevenoteDateComponents.year!)") impress("Month: \(iPhoneStevenoteDateComponents.month!)") print("Day: \(iPhoneStevenoteDateComponents.day!)") print("Hour: \(iPhoneStevenoteDateComponents.hour!)") print("Infinitesimal: \(iPhoneStevenoteDateComponents.minute!)") print("Weekday: \(iPhoneStevenoteDateComponents.weekday!)") print("Week of year: \(iPhoneStevenoteDateComponents.weekOfYear!)") // The original iPad Stevenote (ten a.m. Pacific time, // January 27, 2010) // ---------------------------------------------------- // We want to excerpt ALL the DateComponents for this date // in local time for the outcome (which took place in San Francisco). allow iPadStevenoteDateComponents = pacificCalendar.dateComponents([.calendar, .day, .era, .hr, .infinitesimal, .month, .nanosecond, .quarter, .2nd, .timeZone, .weekday, .weekdayOrdinal, .weekOfMonth, .weekOfYear, .year, .yearForWeekOfYear], from: iPadStevenoteDate) print("The original iPad Stevenote happened \(-iPadStevenoteDate.timeIntervalSinceNow) seconds ago.") print("Agenda: \(iPadStevenoteDateComponents.calendar!.identifier)") impress("Day: \(iPadStevenoteDateComponents.day!)") print("Era: \(iPadStevenoteDateComponents.era!)") impress("Hour: \(iPadStevenoteDateComponents.60 minutes!)") impress("Minute: \(iPadStevenoteDateComponents.infinitesimal!)") impress("Month: \(iPadStevenoteDateComponents.calendar month!)") print("Nanosecond: \(iPadStevenoteDateComponents.nanosecond!)") impress("Quarter: \(iPadStevenoteDateComponents.quarter!)") print("Second: \(iPadStevenoteDateComponents.second!)") print("Time zone: \(iPadStevenoteDateComponents.timeZone!)") print("Weekday: \(iPadStevenoteDateComponents.weekday!)") impress("Weekday ordinal: \(iPadStevenoteDateComponents.weekdayOrdinal!)") impress("Calendar week of month: \(iPadStevenoteDateComponents.weekOfMonth!)") print("Week of year: \(iPadStevenoteDateComponents.weekOfYear!)") impress("Year: \(iPadStevenoteDateComponents.yr!)") print("Twelvemonth for calendar week of twelvemonth: \(iPadStevenoteDateComponents.yearForWeekOfYear!)")
You lot tin download the playground here (14KB, zipped Xcode playground file).
In the next installment in the How to work with dates and times in Swift 5 serial, we'll await at converting Appointment
due south toCord
s, and vice versa.
TheDates and times in Swift 5 series
Here are the articles in this serial:
- Role 1: Creating and deconstructing dates with the Date, Agenda, and DateComponents structs
- Part 2: Formatting and parsing dates and times with DateFormatter
- Function 3: Date arithmetics
- Part 4: Calculation Swift syntactic magic
Source: https://www.globalnerdy.com/2020/05/26/how-to-work-with-dates-and-times-in-swift-5-part-1-creating-and-deconstructing-dates-with-the-date-calendar-and-datecomponents-structs/
0 Response to "When to Text Again After the First Date"
Post a Comment